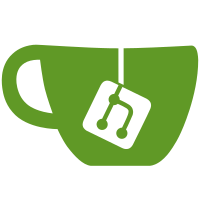
code was modified slightly, so the code differs from the original downloadable 1.9.5 version
98 lines
3.1 KiB
PHP
98 lines
3.1 KiB
PHP
<?php
|
|
/**
|
|
* Zend Framework
|
|
*
|
|
* LICENSE
|
|
*
|
|
* This source file is subject to the new BSD license that is bundled
|
|
* with this package in the file LICENSE.txt.
|
|
* It is also available through the world-wide-web at this URL:
|
|
* http://framework.zend.com/license/new-bsd
|
|
* If you did not receive a copy of the license and are unable to
|
|
* obtain it through the world-wide-web, please send an email
|
|
* to license@zend.com so we can send you a copy immediately.
|
|
*
|
|
* @category Zend
|
|
* @package Zend
|
|
* @subpackage UnitTests
|
|
* @copyright Copyright (c) 2005-2009 Zend Technologies USA Inc. (http://www.zend.com)
|
|
* @license http://framework.zend.com/license/new-bsd New BSD License
|
|
* @version $Id: TestHelper.php 18709 2009-10-26 13:33:53Z matthew $
|
|
*/
|
|
|
|
/*
|
|
* Include PHPUnit dependencies
|
|
*/
|
|
require_once 'PHPUnit/Framework.php';
|
|
require_once 'PHPUnit/Framework/IncompleteTestError.php';
|
|
require_once 'PHPUnit/Framework/TestCase.php';
|
|
require_once 'PHPUnit/Framework/TestSuite.php';
|
|
require_once 'PHPUnit/Runner/Version.php';
|
|
require_once 'PHPUnit/TextUI/TestRunner.php';
|
|
require_once 'PHPUnit/Util/Filter.php';
|
|
|
|
/*
|
|
* Set error reporting to the level to which Zend Framework code must comply.
|
|
*/
|
|
error_reporting( E_ALL | E_STRICT );
|
|
|
|
/*
|
|
* Determine the root, library, and tests directories of the framework
|
|
* distribution.
|
|
*/
|
|
$zfRoot = dirname(__FILE__) . '/..';
|
|
$zfCoreLibrary = "$zfRoot/library";
|
|
$zfCoreTests = "$zfRoot/tests";
|
|
|
|
/*
|
|
* Omit from code coverage reports the contents of the tests directory
|
|
*/
|
|
foreach (array('php', 'phtml', 'csv') as $suffix) {
|
|
PHPUnit_Util_Filter::addDirectoryToFilter($zfCoreTests, ".$suffix");
|
|
}
|
|
|
|
/*
|
|
* Prepend the Zend Framework library/ and tests/ directories to the
|
|
* include_path. This allows the tests to run out of the box and helps prevent
|
|
* loading other copies of the framework code and tests that would supersede
|
|
* this copy.
|
|
*/
|
|
$path = array(
|
|
$zfCoreLibrary,
|
|
$zfCoreTests,
|
|
get_include_path()
|
|
);
|
|
set_include_path(implode(PATH_SEPARATOR, $path));
|
|
|
|
/*
|
|
* Load the user-defined test configuration file, if it exists; otherwise, load
|
|
* the default configuration.
|
|
*/
|
|
if (is_readable($zfCoreTests . DIRECTORY_SEPARATOR . 'TestConfiguration.php')) {
|
|
require_once $zfCoreTests . DIRECTORY_SEPARATOR . 'TestConfiguration.php';
|
|
} else {
|
|
require_once $zfCoreTests . DIRECTORY_SEPARATOR . 'TestConfiguration.php.dist';
|
|
}
|
|
|
|
/**
|
|
* Start output buffering, if enabled
|
|
*/
|
|
if (defined('TESTS_ZEND_OB_ENABLED') && constant('TESTS_ZEND_OB_ENABLED')) {
|
|
ob_start();
|
|
}
|
|
|
|
/*
|
|
* Add Zend Framework library/ directory to the PHPUnit code coverage
|
|
* whitelist. This has the effect that only production code source files appear
|
|
* in the code coverage report and that all production code source files, even
|
|
* those that are not covered by a test yet, are processed.
|
|
*/
|
|
if (defined('TESTS_GENERATE_REPORT') && TESTS_GENERATE_REPORT === true &&
|
|
version_compare(PHPUnit_Runner_Version::id(), '3.1.6', '>=')) {
|
|
PHPUnit_Util_Filter::addDirectoryToWhitelist($zfCoreLibrary);
|
|
}
|
|
|
|
/*
|
|
* Unset global variables that are no longer needed.
|
|
*/
|
|
unset($zfRoot, $zfCoreLibrary, $zfCoreTests, $path);
|